Chess using Python3
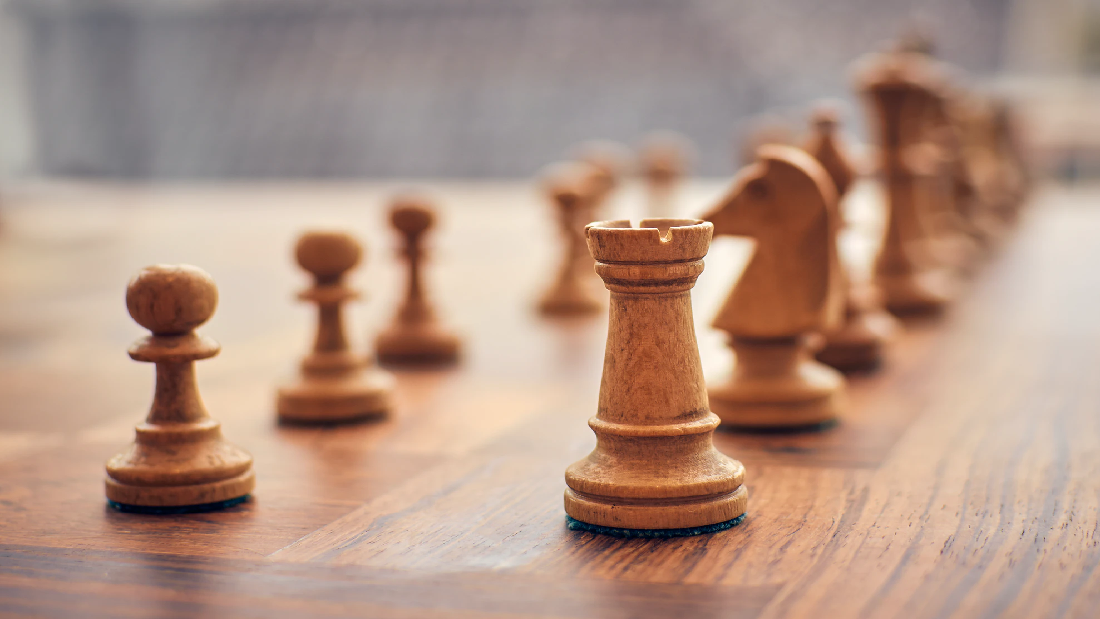
Chess is among the most popular board games on Earth, with a history of over thousands of years. It has also been one of the most widely studied games in the domain of AI, due to its strategic nature. This has lead to the development of many advanced chess engines which has the ability to even beat world champions (Deep Blue versus Garry Kasparov, 1997).
Today, I want to discuss about a python library I found which could visualize chess boards, help identify the possible legal moves etc. It also has the ability to integrate with chess engines like Stockfish to make intelligent moves as well as analyze the board position provided to it.
Let us first install the chess library using pip command:
pip install chess
Once you have successfully installed the chess library, you will be able to import it into your python program using import command. Our first objective is to play a simple game where we provide the program with all the moves. We will be implementing scholar’s mate (shown in Figure 1).
import chess
board = chess.Board()
moves = ["e4","e5","Qh5","Nc6","Bc4","Nf6","Qf7"]
for i in moves:
board.push_san(i)
print(board.is_game_over())
board
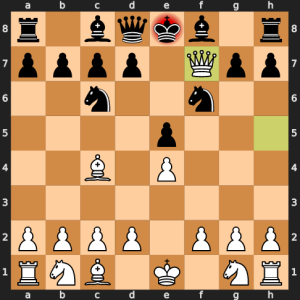
Let us now try to read an existing game using our chess library, for this example we will be considering the game 6 between Bobby Fischer and Boris Spassky in 1972 world chess championship. PGN is a standard readable format in which chess games are saved in computer.
import chess.pgn as PGN
#Game pgn taken from chessgames.com
with open("fischer_spassky_1972.pgn") as pgn:
first_game = PGN.read_game(pgn)
moves = str(first_game.mainline())
print("White Player:",first_game.headers["White"])
print("Black Player:",first_game.headers["Black"])
print("Game Mainline:\n"+moves)
print("Result:",first_game.headers["Result"])
Using help(chess.board) you will able to see the wide array of functions that have been implemented in this chess library. Let us now see how we can integrate stockfish, a famous open source chess engine with our python chess library. For this first we need to have stockfish application available in our system and it can be downloaded here. We will be import the stockfish application and let it play against itself. We will visualize the board in ascii form at each step and then visualize the final board position.
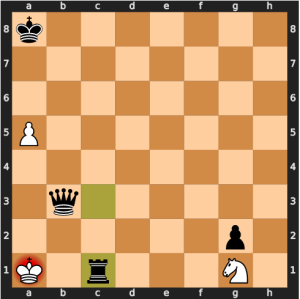
In this blogpost we saw the working of chess library in python, however we were only able to see some of the basic functions provided in this library. Do refer to the original documentation to get a more in-depth idea of this library and its functionality. You can find the code for this blogpost in this github repo.